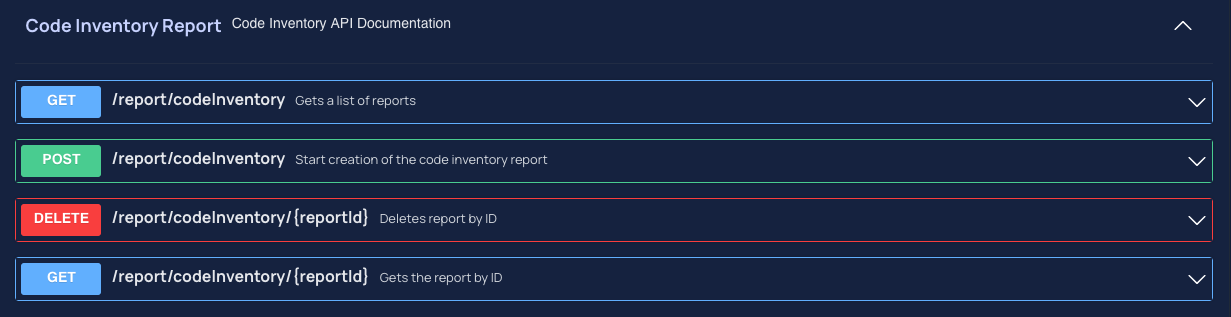
API for Code Inventory
The Code Inventory API allows you to create and download reports to get insights into how your Java code is used in production.
Reports can be grouped by JAR, classes and/or methods.
Reports are generated asynchronously. By using the endpoint, a task to generate a report is created. In response, you get a unique report identifier. With this identifier, you can retrieve the report.
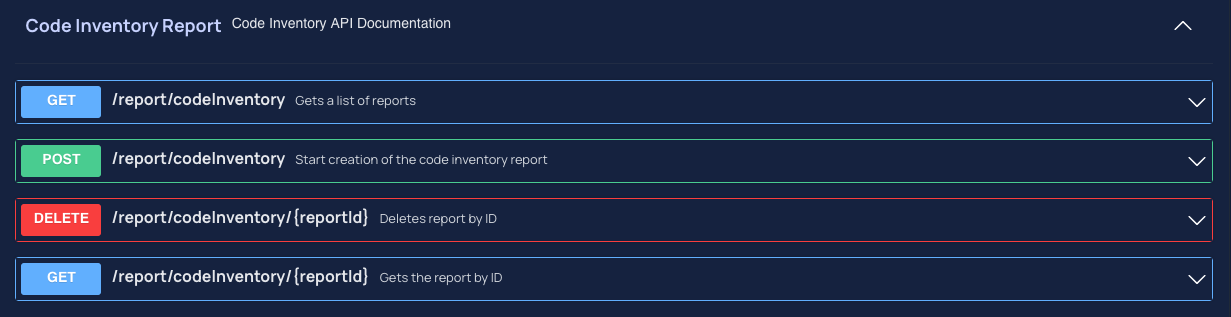
Request Report Generation
You can request the generation of a report with a POST
call to https://YOUR_ENDPOINT.azul.com/public/report/codeInventory
and a set of parameters.
Request Parameters
Argument | Default | Description |
---|---|---|
filter |
array[string] |
Array of filters for one or more of the following (case-sensitive) attributes:
Usage example:
The operator |
from |
string |
Relative or absolute time for the start of the report. See API Date Filter Format. |
to |
string |
Relative or absolute time for the end of the report. See API Date Filter Format. |
Example Request
curl -X 'POST' \
'https://YOUR_ENDPOINT.azul.com/report/codeInventory' \
-H 'accept: application/json' \
-H 'Content-Type: application/json' \
-H 'x-api-key: YOUR_API_KEY' \
-d '{
"filter": [
"sourceName CONTAINS 'myapp.jar'",
"className CONTAINS 'com/company/FirstClass'",
"methodName CONTAINS 'myMethod'"
],
"from": "2024-10-14T03:46:00.000+0200",
"to": "2024-10-15T03:59:59.999+0200"
}'
Example Response
In response, you get a reportId that can be used with the GET
endpoint to retrieve the report.
{
"reportId": "d0ce659c-e71e-4aea-b5a7-b817482c4ac9",
"state": "PROCESSING",
"stateMessages": [
"1st message",
"2nd message"
],
"userId": "[email protected]",
"params": {
"filter": [
"sourceName CONTAINS 'myapp.jar'",
"className CONTAINS 'com/company/FirstClass'",
"methodName CONTAINS 'myMethod'"
],
"from": "2024-10-14T03:46:00.000+0200",
"to": "2024-10-15T03:59:59.999+0200",
"resolvedFrom": "2024-10-14T03:46:00.000+0200",
"resolvedTo": "2024-10-15T03:59:59.999+0200"
},
"requestTime": "2024-10-16T00:00:00.000Z"
}
You can check the resolvedFrom
and resolvedTo
values that are used for the creation of the report, in case you provided relative timestamps in the request:
{
...
"params": {
"filter": [
...
],
"from": "3m",
"to": "0d",
"resolvedFrom": "2024-05-16T00:00:00.000",
"resolvedTo": "2024-08-16T23:59:59.999"
},
...
}
Retrieve the Report
You can retrieve a report with a GET
call to /public/report/codeInventory/YOUR_REPORT_ID
.
The response must be checked to define if the data is available, based on the following possible return data:
-
state
: State of the request, one of the following options:-
PROCESSING
-
SUCCEEDED
-
FAILED
-
PARTIALLY_FAILED
-
TIMED_OUT
-
-
stateMessages
: More info related to thestate
. -
errors
: Errors while creating the report (if applicable).
Large reports are split into pages (up to 5.5MB per page). You can find the total number of pages in the response and use this value to retrieve all the report pages.
Note
|
Regardless of whether a report is generated successfully or not, when the generation is finished, at least one page of the report is available for retrieval. |
Example Request
curl -X 'GET' \
'https://YOUR_ENDPOINT.azul.com/public/report/codeInventory/YOUR_REPORT_ID' \
-H 'accept: application/json' \
-H 'x-api-key: YOUR_API_KEY' \
-H 'Content-Type: application/json'
Example Response
{
"reportId": "d0ce659c-e71e-4aea-b5a7-b817482c4ac9",
"reportName": "demo_report",
"userId": "[email protected]",
"params": {
"filter": [
"sourceName CONTAINS 'myapp.jar'",
"className CONTAINS 'com/company/FirstClass'",
"methodName CONTAINS 'myMethod'"
],
"from": "2024-10-14T03:46:00.000+0200",
"to": "2024-10-15T03:59:59.999+0200",
"resolvedFrom": "2024-10-14T03:46:00.000",
"resolvedTo": "2024-10-15T03:59:59.999"
},
"state": "SUCCEEDED",
"requestTime": "2024-10-15T03:47:36.472",
"startTime": "2024-10-15T03:47:36.594",
"finishTime": "2024-10-15T03:47:37.352",
"totalCount": 2,
"data": [
{
"sourceName": "myapp.jar",
"className": "com/company/firstclass",
"methodName": "mymethod(java/lang/String)",
"firstSeen": "2024-10-02T12:37:46.989",
"lastSeen": "2024-10-10T11:02:23.371",
"AppEnv": "UNDEFINED"
},
{
"sourceName": "myapp.jar",
"className": "com/company/firstclass",
"methodName": "mymethod(java/lang/String)",
"firstSeen": "2024-10-10T12:37:46.989",
"lastSeen": "2024-10-16T11:02:23.371",
"AppEnv": "UNDEFINED"
}
]
}
When the JMV is configured with the AppEnv
tag, as described here, the data block includes that extra info. For example:
{
"reportId": "d0ce659c-e71e-4aea-b5a7-b817482c4ac9",
...
"data": [
{
"sourceName": "myapp.jar",
"className": "com/company/FirstClass",
"methodName": "myMethod(java/lang/String)",
"firstSeen": "2024-10-02T12:37:46.989",
"lastSeen": "2024-10-10T11:02:23.371",
"AppEnv": "your_tag"
},
...
],
...
}
Retrieve All Reports
You can retrieve a list with all reports with a GET
call to https://YOUR_ENDPOINT.azul.com/public/report/codeInventory
.
curl -X 'GET' \
'https://YOUR_ENDPOINT.azul.com/public/report/codeInventory' \
-H 'accept: application/json' \
-H 'x-api-key: YOUR_API_KEY' \
-H 'Content-Type: application/json'
Example Response
{
"data": [
{
"reportId": "1cc40ee6-87a7-4147-b02d-7d26ce0387d0",
"state": "SUCCEEDED",
"stateMessages": [
"1st message",
"2nd message"
],
"userId": "[email protected]",
"params": {
"filter": [
"sourceName CONTAINS 'myapp.jar'",
"className CONTAINS 'com/company/FirstClass'",
"methodName CONTAINS 'myMethod'"
],
"from": "2023-02-15T00:00:00.000+0200",
"to": "2023-02-16T23:59:59.999+0200",
"resolvedFrom": "2023-02-15T00:00:00.000+0200",
"resolvedTo": "2023-02-16T23:59:59.999+0200"
},
"requestTime": "2023-02-15T00:00:00.000Z",
"startTime": "2023-02-15T00:00:00.000Z",
"finishTime": "2023-02-15T00:00:00.000Z"
}
],
"afterToken": "1683200412"
}
Delete a Report
You can delete a report with a DELETE
call to https://YOUR_ENDPOINT.azul.com/public/report/codeInventory/YOUR_REPORT_ID
.